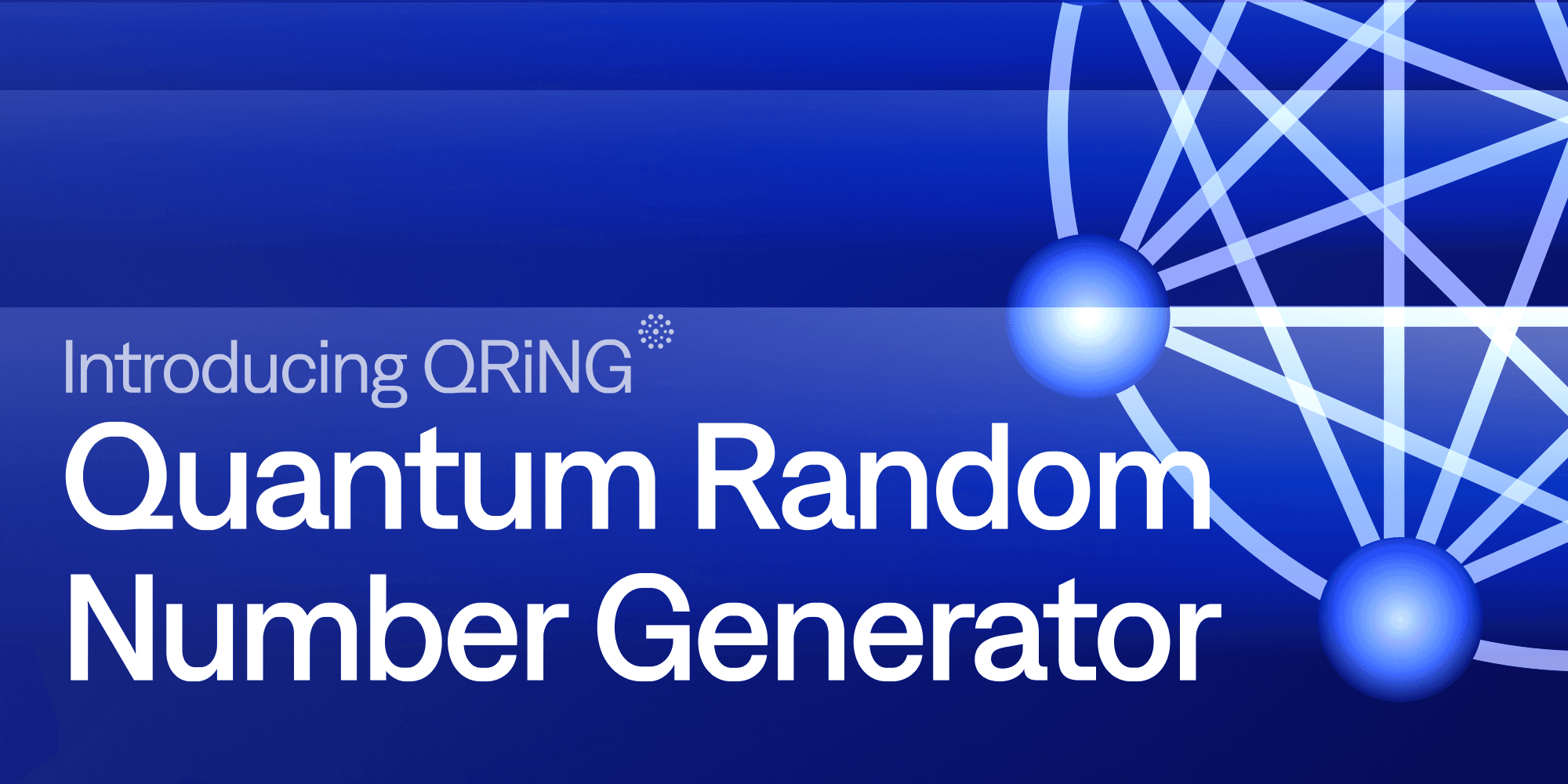
QRiNG
QRiNG is a Quantum Random Number Generator (QRNG) that generates random numbers using quantum mechanical processes. Unlike classical random number generators, which rely on algorithms and are inherently predictable, QRNGs exploit the fundamental unpredictability of quantum phenomena to produce truly random numbers.
Quantum Consensus Networks (QCNs):
Quantum Consensus Networks (QCNs) are a framework that leverages quantum-enabled nodes to form consensus on the generation of certifiable quantum randomness. Quantum hardware in these networks can utilize the randomness provided by dedicated QCNs. In our protocol, Quantum Key Distribution (QKD) is used to generate random numbers via pairwise node operations.
Quantum Key Distribution (QKD):
Quantum Key Distribution (QKD) enables the secure establishment of shared randomness between two parties with information-theoretic security. This protocol is resistant to spoofing when executed honestly.
Referring to the well-known BB84 protocol, we assume that all node pairs in the network have access to an unlimited supply of maximally entangled Bell pairs:







Consensus Protocol


Implementation using Smart Contract:

// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract SmartContract {
struct Voter {
address delegate;
bool hasVoted;
uint number;
uint voteCount;
uint[] bitstring;
}
Voter[] public voters;
address public admin;
bool public votingActive;
uint[][] public counter;
event VoterRegistered(address voter);
event VotingEnded();
modifier onlyAdmin() {
require(msg.sender == admin, "Only admin can call this function");
_;
}
modifier onlyActive() {
require(votingActive, "Voting is not active");
_;
}
function addNewString(uint[][] memory newString) public {
counter = newString;
}
function setAddresses(address[] memory voterAddresses) public {
admin = msg.sender;
votingActive = true;
for (uint x = 0; x < voterAddresses.length; x++) {
voters.push(Voter({
delegate: voterAddresses[x],
hasVoted: false,
number: x,
voteCount: 0,
bitstring: counter[x]
}));
emit VoterRegistered(voterAddresses[x]);
}
}
function startVoting() external onlyAdmin {
require(!votingActive, "Voting is already active");
votingActive = true;
}
function endVoting() external onlyAdmin {
require(votingActive, "Voting is not active");
votingActive = false;
emit VotingEnded();
}
function check(uint from) external onlyActive {
require(voters[from].delegate == msg.sender, "Not authorized");
require(!voters[from].hasVoted, "Voter has already voted");
uint bitstringLength = voters[from].bitstring.length / 2;
for (uint i = 0; i < voters.length; i++) {
if (from != i) {
uint add = 0;
for (uint j = 0; j < voters[from].bitstring.length; j++) {
if (voters[from].bitstring[j] == voters[i].bitstring[j]) {
add++;
}
}
if (add > bitstringLength) {
voters[i].voteCount++;
}
}
}
voters[from].hasVoted = true;
}
function getWinner() external view returns (uint8) {
require(!votingActive, "Voting is still active");
uint8 sum = 0;
uint256 len = voters.length / 2;
for (uint i = 0; i < voters.length; i++) {
if (voters[i].voteCount > len) {
sum++;
}
}
return sum;
}
function randomNumber() external view returns (uint[6] memory newBitstring) {
require(!votingActive, "Voting is still active");
uint256 len = voters.length / 2;
for (uint i = 0; i < voters.length; i++) {
if (voters[i].voteCount > len) {
for (uint x = 0; x < voters[i].bitstring.length; x++) {
newBitstring[x] += voters[i].bitstring[x];
newBitstring[x] %= 2;
}
}
}
return newBitstring;
}
}
In this contract, nodes vote on each others’ quantum validity using bitstrings following the QKD protocol. Here’s a breakdown of the contract:
- The contract is owned by the address specified during deployment.
- The contracts re quires the bitstrings as well as the addresses of the participating accounts. The bitstrings are then allotted to each node in the network.
- The add_new_string function allows the admin to add bitstrings ($s_{i,j}$).
- The set_addresses function inputs a list of addresses and initializes other parameters in the Voter struct.
- The CHECK function acts as the certification function, comparing various bitstrings .
- The get_Winner function is used to determine the node (address) with the highest number of votes.
- The random_number function implements a condition that decides the honesty of nodes based on majority votes. Once the honest nodes are determined, an XOR operation on bitstrings of these nodes is calculated. This is the random number generated at the end of the protocol.
- The modifiers onlyActive() and onlyAdmin() are used as constraints to ensure functions in the smart contract are only run on certain conditions. onlyActive() ensures that the function is run while the voting is active, while onlyAdmin() is used to control the voting by the admin.
- The StartVoting() and EndVoting() functions are called by the admin to allow voting to take place and subsequently, generate the random number.
Simulation results
We conduct simulations on our QRiNG protocol by using the same logic in a python script. We look at asymptotic results for such a protocol with fixed number of nodes and bitstrings.


To inquire or learn more about QRiNG please visit https://www.btq.com/products/qring